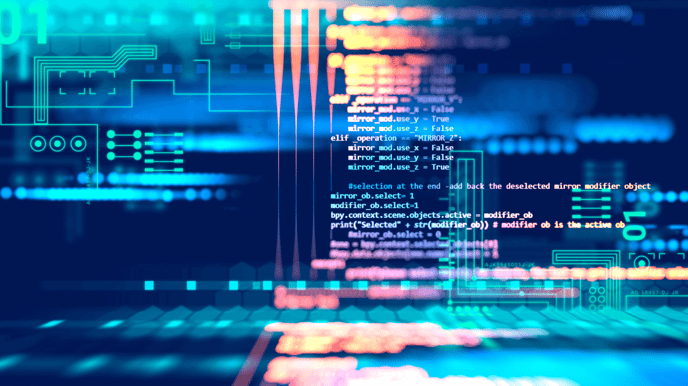
Roblox Lua Scripting: Getting Started with Coding
Posted by Emily Carson October 26th, 2024
Getting started with coding in Roblox can feel like learning a new language—because, well, it is! Roblox uses Lua, a scripting language known for its simplicity and versatility, making it a perfect introduction to coding for both beginners and aspiring game developers. Lua is the magic that brings Roblox games to life, letting creators control how players interact with the world, create custom actions, and even design entire minigames. Here’s a guide to getting your feet wet in Lua scripting and unlocking the creative possibilities that come with it.
Step One: Opening Roblox Studio and the Script Editor
Before you can start coding, you need Roblox Studio, the platform where all the magic happens. If you haven’t downloaded it yet, you can get it from the Roblox website. Once in Roblox Studio, create a new game or open an existing one, and get ready to dive into Lua.
To start scripting, go to the Explorer panel on the right side of Roblox Studio. Right-click on the part or object you want to code for, select “Insert Object,” and then choose “Script.” This opens a blank script window where you’ll write your code. Think of it as your canvas; every line you type here will define how the selected object behaves.
Step Two: Writing Your First Line of Code
Let’s start small. One of the simplest commands in Lua is print(), which is often used to display text in the output window. It’s a great first step, a way to check that your script is working.
In the script window, type:
print("Hello, Roblox!")
When you press “Play” in Roblox Studio, this command will display “Hello, Roblox!” in the output window, confirming that your script is active. It may seem simple, but this is your first interaction with Lua. Every code journey begins with a single line, and this one is yours.
Step Three: Adding Interaction with Functions
Now that you’re comfortable with the basics, it’s time to make your code interactive. Functions in Lua are blocks of code that perform specific tasks when called upon. Let’s create a simple function that will make an object change color when clicked.
Here’s a basic example:
function changeColor()
script.Parent.BrickColor = BrickColor.Random()
end
script.Parent.ClickDetector.MouseClick:Connect(changeColor)
In this code:
• changeColor() is a function that changes the color of the object to a random color.
• script.Parent refers to the object your script is attached to.
• ClickDetector.MouseClick triggers the changeColor function when the object is clicked.
To make this code work, make sure to add a ClickDetector to the object in Roblox Studio. Now, when you run the game and click on the object, it will change colors—a simple but satisfying interaction that makes your game world feel dynamic.
Step Four: Exploring Variables and Events
Variables are essential to coding, as they store information that you can use throughout your script. Let’s say you want to control the speed of an object. You can create a variable that stores the speed and then use it to control the object’s movement.
Here’s an example:
local speed = 10
function movePart()
script.Parent.Position = script.Parent.Position + Vector3.new(0, speed, 0)
end
script.Parent.ClickDetector.MouseClick:Connect(movePart)
In this script:
• local speed = 10 defines a variable called speed and sets it to a value of 10.
• Vector3.new(0, speed, 0) moves the object upward by the value of speed.
With each click, the object will move up, thanks to the function triggered by MouseClick. This is just the beginning—variables and events give you flexibility and control, letting you manage almost any action in your game.
Step Five: Adding Conditional Statements
In coding, conditions help make decisions. With Lua, you can use if statements to control the behavior of your game based on certain conditions. Let’s say you want an object to change color only if it’s a certain color.
Here’s how you’d do it:
function checkColor()
if script.Parent.BrickColor == BrickColor.new("Bright red") then
script.Parent.BrickColor = BrickColor.new("Bright blue")
else
script.Parent.BrickColor = BrickColor.new("Bright red")
end
end
script.Parent.ClickDetector.MouseClick:Connect(checkColor)
In this example:
• The if statement checks if the object’s color is bright red. If it is, the color changes to bright blue. Otherwise, it changes to red.
• Conditional statements add logic, allowing your game to respond differently based on the situation. This is powerful because it allows for branching behaviors, adding depth to player interactions.
Step Six: Testing and Debugging
Once you’ve written some scripts, it’s essential to test them. Roblox Studio offers a Play mode where you can experience your game as a player would. Test each feature, interact with objects, and check that everything works as intended.
Sometimes, scripts won’t work the way you expect. This is where debugging comes in. Use print() statements to display values or messages in the output window, helping you track down issues. For example, if a function isn’t working, add print(“Function triggered”) to confirm if it’s even being called. Debugging can be a challenge, but it’s an invaluable part of learning to code.
Taking the Next Steps in Lua
Getting started with Lua in Roblox is just the beginning. As you grow more comfortable, you’ll be able to create complex systems—everything from custom animations to entire game mechanics. There are endless resources and communities where you can learn more, ask questions, and share your progress. Keep experimenting, keep coding, and remember that every line brings you closer to bringing your Roblox ideas to life.
Roblox Lua scripting is more than just coding; it’s a gateway into game development, a tool that transforms ideas into actions. As you continue exploring, you’ll find that Roblox isn’t just a game—it’s a place where you can build, learn, and create something truly your own.